Tailwind: Because Life's Too Short for !important
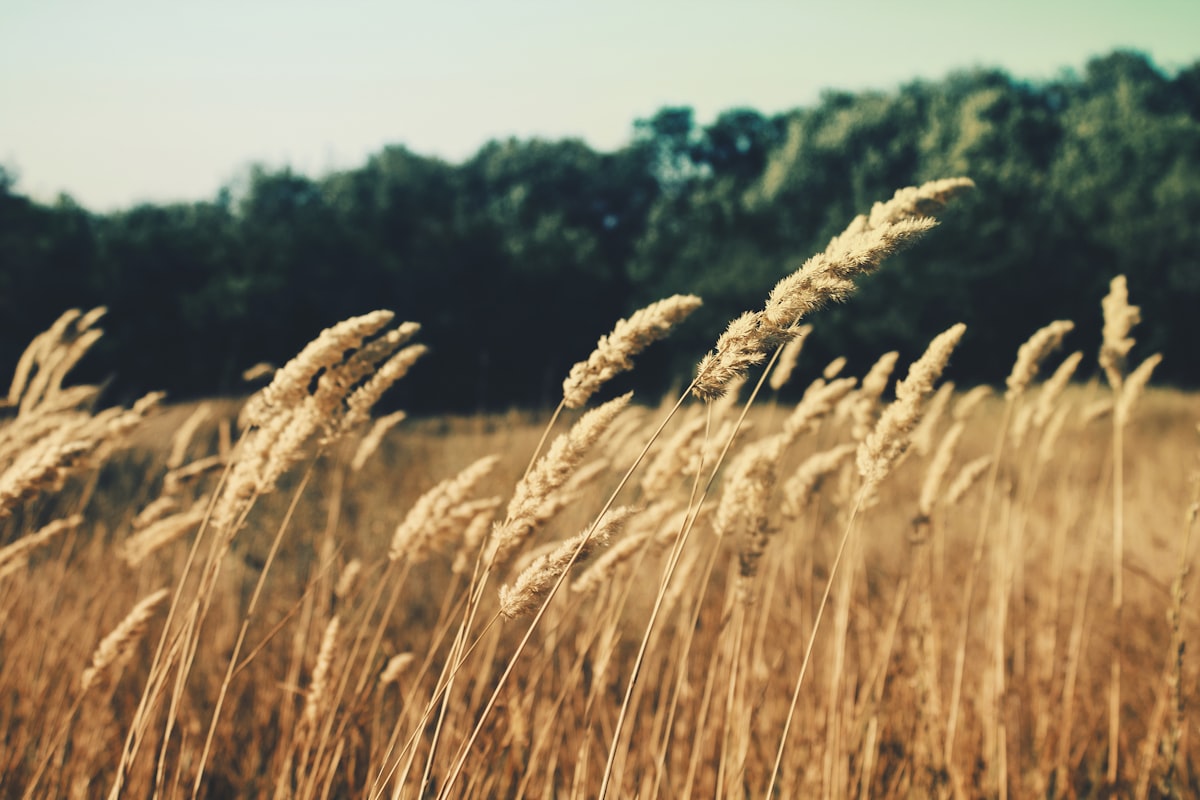
CSS frameworks have become a hot topic in front-end development in recent years. Tailwind CSS has emerged as a noteworthy contender, separating the developer community from either love or hate for Tailwind.
In this article, I will talk about the advantages of Tailwind CSS compared to traditional CSS, focusing on how it streamlines the development process. I'll use SCSS examples to illustrate key points and demonstrate why Tailwind has gained popularity among many developers.
Whether you're a CSS newbie, a seasoned front-end engineer, or a back-end engineer who breaks out in a cold sweat at the mere mention of CSS, this will be useful as I will try to provide valuable reasons why writing CSS code with Tailwind is not so scary.
What is Tailwind?
We all know simple CSS or SCSS, so imagine you want to style a simple button with a nice hover effect.
You might style the button using nested rules and variables:
$primary-color: #3b82f6;
$text-color: #ffffff;
$font-weight: 700;
$padding-y: 0.5rem;
$padding-x: 1rem;
$border-radius: 0.25rem;
.primary-button {
background-color: $primary-color;
color: $text-color;
font-weight: $font-weight;
padding: $padding-y $padding-x;
border-radius: $border-radius;
&:hover {
background-color: darken($primary-color, 10%);
}
}
Instead of writing custom CSS for a button, you might use Tailwind classes like this:
<button class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
Click me
</button>
You probably think "Did a cat just walk across your keyboard?"
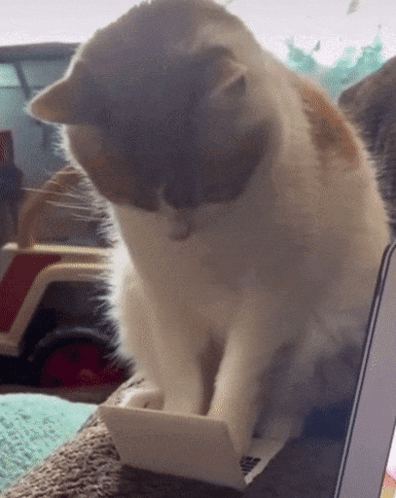
but the Tailwind classes directly applied to the HTML element provide the following:
bg-blue-500
: Sets the background color (equivalent to#3b82f6
)hover:bg-blue-700
: Changes the background on hover (similar to the SCSSdarken
function)text-white
: Sets the text color to whitefont-bold
: Makes the text bold (equivalent tofont-weight: 700
)py-2 px-4
: Adds padding (y-axis: 0.5rem, x-axis: 1rem)rounded
: Applies border-radius for rounded corners
So, we can say that Tailwind is a utility-first CSS framework that provides pre-defined classes to style elements directly in your HTML.
Here are some reasons why I prefer Tailwind over standard CSS frameworks.
1) Say goodbye to the class-naming marathon
In the example above, Tailwind classes like bg-blue-500
, hover:bg-blue-700
, and font-bold
are used to style the button. These classes are descriptive and easy to understand, making the code more readable and maintainable.
With SCSS, you create a custom class name .button-primary
and define its styles in a separate SCSS file. While this approach allows for more flexibility, it requires you to think of meaningful class names and maintain them throughout your project.
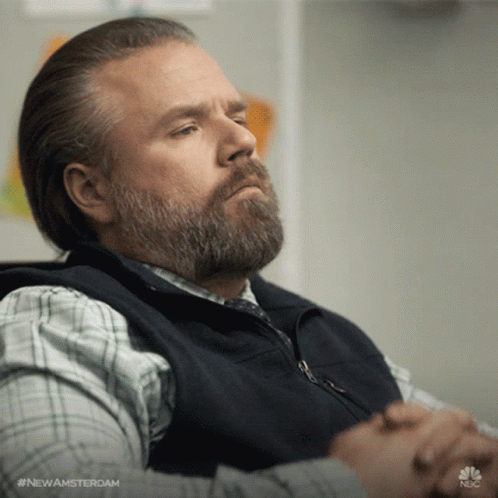
For me, this is a big plus for Tailwind, as it provides a standardized set of classes, which provides consistent styling across your project.
Tailwind classes are descriptive and self-explanatory, making it easy to understand what styles are applied to an element. With VS Code Tailwind extension you can see real CSS by hovering over the Tailwind class.
You don't need to worry about creating and maintaining custom class names. Just apply the pre-defined utility classes to your elements.
2) Say hello to lean, mean CSS
When it comes to generating CSS, Tailwind and SCSS take different approaches.
Tailwind automatically generates the smallest amount of CSS necessary based on the utility classes used in your HTML, while SCSS compiles all the styles defined in your .scss
files, regardless of whether they're used or not.
Tailwind generates CSS on-demand by scanning your HTML files and generating only the styles that are actually used.
Consider the following example:
<div class="bg-blue-500 text-white p-4">
<h1 class="text-2xl font-bold mb-2">Welcome!</h1>
<p class="text-lg">This is a Tailwind example.</p>
</div>
When you run Tailwind's build process, it analyzes the HTML and generates a minimal CSS file containing only the styles used in the markup:
.bg-blue-500 { background-color: #3b82f6; }
.text-white { color: #fff; }
.p-4 { padding: 1rem; }
.text-2xl { font-size: 1.5rem; }
.font-bold { font-weight: 700; }
.mb-2 { margin-bottom: 0.5rem; }
.text-lg { font-size: 1.125rem; }
In contrast, SCSS compiles all the styles defined in your .scss
files, even if they're not used in your HTML.
Consider the following example:
.container {
background-color: #3b82f6;
color: #fff;
padding: 1rem;
h1 {
font-size: 1.5rem;
font-weight: 700;
margin-bottom: 0.5rem;
}
p {
font-size: 1.125rem;
}
}
.unused-class {
background-color: #f00;
padding: 2rem;
}
When you compile this SCSS file, it generates a CSS file containing all the defined styles, including the unused .unused-class
:
.container {
background-color: #3b82f6;
color: #fff;
padding: 1rem;
}
.container h1 {
font-size: 1.5rem;
font-weight: 700;
margin-bottom: 0.5rem;
}
.container p {
font-size: 1.125rem;
}
.unused-class {
background-color: #f00;
padding: 2rem;
}
By generating only the styles that are actually used, Tailwind produces smaller CSS files, reducing the amount of data transferred to the browser.
Smaller CSS files lead to faster page load times, improving the user experience. With Tailwind, you don't have to worry about removing unused styles manually, as they're never generated in the first place.
3) Transform design into reality in the blink of an eye
When it comes to implementing custom designs, Tailwind and SCSS offer different approaches that can impact your development speed.
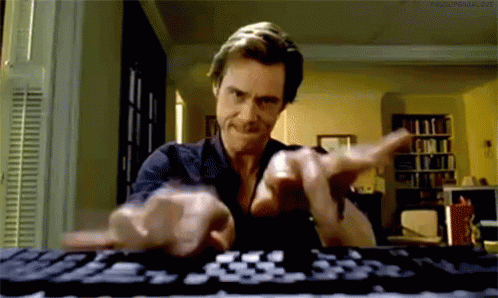
Tailwind's utility-first approach allows you to rapidly build custom layouts and components, while SCSS requires more setup and custom styling.
Tailwind's set of utility classes allows you to build custom designs really fast. Consider the following example of a custom card component:
<div class="bg-white rounded-lg shadow-md p-6">
<img class="w-full h-48 object-cover mb-4" src="card-image.jpg" alt="Card Image">
<h2 class="text-xl font-bold mb-2">Card Title</h2>
<p class="text-gray-700">This is a custom card component built with Tailwind utility classes.</p>
<button class="bg-blue-500 text-white px-4 py-2 rounded-md mt-4">Read More</button>
</div>
In this example, we use Tailwind utility classes like bg-white
, rounded-lg
, shadow-md
, and p-6
to style the card container.
The image, title, description, and button are also styled using Tailwind classes, allowing us to create a custom design without writing any custom CSS.
With SCSS, you define custom styles for each component and set up the necessary HTML structure. Here's an example of the same card component using SCSS:
.card {
background-color: #fff;
border-radius: 0.5rem;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
padding: 1.5rem;
&__image {
width: 100%;
height: 12rem;
object-fit: cover;
margin-bottom: 1rem;
}
&__title {
font-size: 1.25rem;
font-weight: bold;
margin-bottom: 0.5rem;
}
&__description {
color: #4a5568;
}
&__button {
background-color: #3b82f6;
color: #fff;
padding: 0.5rem 1rem;
border-radius: 0.375rem;
margin-top: 1rem;
}
}
and this is the HTML code:
<div class="card">
<img class="card__image" src="card-image.jpg" alt="Card Image">
<h2 class="card__title">Card Title</h2>
<p class="card__description">This is a custom card component built with SCSS.</p>
<button class="card__button">Read More</button>
</div>
With SCSS, you need to define the styles for each component separately and ensure that the HTML structure matches the CSS selectors. This process can take more time compared to using Tailwind's pre-built utility classes.
With Tailwind, you can build custom components and layouts quickly by combining utility classes directly in your HTML. Pre-defined classes ensure consistent styling across your project, making it easier to maintain a cohesive design.
4) Say goodbye to the domino effect of cascading-style disasters
When working on large projects with multiple developers, it's important to ensure that making changes in one part of the project doesn't unintentionally break styles elsewhere.
Tailwind and SCSS handle this concern differently, with Tailwind offering a safer approach to avoiding style conflicts.
Tailwind's utility classes are highly specific and are applied directly to the HTML elements. This means the styles are localized to the specific component or element you're working on.
Consider the following example:
<div class="bg-blue-500 text-white p-4">
<h1 class="text-2xl font-bold mb-2">Welcome!</h1>
<p class="text-lg">This is a Tailwind example.</p>
</div>
In this case, the styles applied using Tailwind classes like bg-blue-500
, text-white
, and p-4
are specific to this particular div
and its children. Changes made here won't affect other parts of the project that use similar classes.
With SCSS, styles are defined in .scss
files and have a global scope. This means that changes in one part of the project can unintentionally affect styles elsewhere.
Consider the following example:
.button {
background-color: #3b82f6;
color: #fff;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
}
If you later decide to change the .button
styles in your SCSS file, it will affect all the buttons throughout your project that use the .button
class.
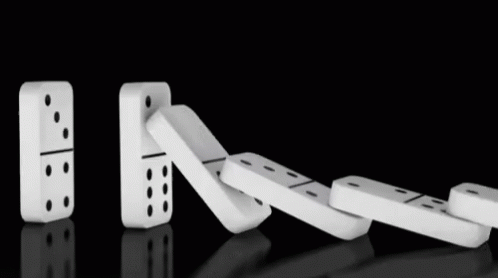
This can lead to unintended consequences and may require you to carefully review and update styles across the entire project.
Tailwind offers several benefits in this case.
By applying styles directly to the elements using specific utility classes, you minimize the risk of accidentally affecting styles elsewhere in the project. You can change the component's styling without worrying about the impact on other parts of the project, making maintenance more straightforward.
It allows multiple developers to work on different components simultaneously without the risk of conflicting styles, enhancing collaboration and reducing merge conflicts.
Verdict
In conclusion, when styling modern web applications, Tailwind CSS stands out as a powerful and efficient choice.
Its utility-first approach and pre-defined classes make it incredibly easy to achieve consistent and responsive designs across your project. With Tailwind, you can rapidly build user interfaces by composing utility classes directly in your HTML, reducing the need for custom CSS.
The Tailwind configuration file allows for seamless customization of settings, base colors, and other design aspects, ensuring that your project aligns perfectly with your unique design system.
Class default values and intuitive naming conventions make it accessible to developers of all skill levels, enabling teams to collaborate effectively and maintain a consistent coding style.
Moreover, Tailwind's performance-focused design ensures that your final CSS bundle remains lean and optimized, leading to faster load times and improved user experiences.
While I like SCSS as it offers some advantages, my favorite is Tailwind. It's simplicity, flexibility, and productivity-boosting features make it a top choice for modern web development.
Comments ()