3 ways to optimize conditional rendering in React
In this short article, we will talk about optimizing conditional rendering code in React and some general good practices.
Through the years I have seen some bad examples that make your code buggy, but also unreadable.
So we can start with first good practice.
Avoid unnecessary ternary operators
Let's say you want to display some content on your page depending on user status. If the user is logged in, you want to display it, otherwise no.
People often do something like this:
isLoggedIn ? <div>Hello {user.fullName}!</div> : null
In this case, there is no need for a ternary operator for such a simple condition. Instead, you can use simple and
operator. Some people also call it a short circuit evaluation.
So you can do something like this:
isLoggedIn && <div>Hello {user.fullName}!</div>
Much better.
Prevent unwanted rendering
Let's say you want to display the number of available items of shop product. People often do something like this:
availableItemsCount && <div>{availableItemsCount} items available!</div>
While at first glance, this code looks ok, it can result in unwanted rendering if the value of cartItemsCount
variable is zero.
React skips rendering for anything that is boolean
or undefined
and will render anything that is string
or number
.
In these cases, it's always good practice to convert the value used in the condition to a boolean and then compare it:
Boolean(availableItemsCount) && <div>{availableItemsCount} items available!</div>
Alternatively, you can also make an explicit number comparison to avoid unwanted rendering:
availableItemsCount > 0 && <div>{availableItemsCount} items available!</div>
With this, you are safe from unwanted renderings.
Avoid (nested) ternary operators
Ternary operators in JSX code are often hard to read. Nested ternaries are even worse.
Take a look at this ugly nested ternary expression:
return isConnected ? (
isWifi ? (
<div>Connected via WiFi</div>
) : (
<div>Connected via mobile data</div>
)
) : (
<div>Not connected</div>
);
When you read code like this, it takes some time to understand what's going on there.
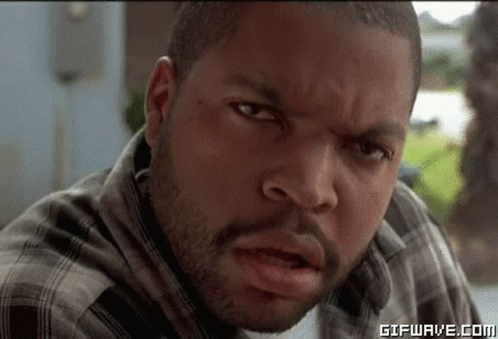
Instead, here is a better approach:
{!isConnected && <div>Not connected</div>}
{isConnected && isWiFi && <div>Connected through WiFi!</div>}
{isConnected && !isWiFi && <div>Connected through mobile data!</div>}
With this, it's far easier to read and understand it.
This is ok code, but I am a fan of early returns so you can take it one step forward and do this in the component code:
const displayConnection = ({isConnected, isWifi}) => {
if(!isConnected){
return <div>Not connected!</div>;
}
if(isWiFi){
return <div>Connected through WiFi!</div>;
}
return <div>Connected through mobile data!</div>
}
With this approach, you also shortened the condition code and removed many &&
operators.
Conclusion
To enhance code readability and maintainability, use logical &&
operators for simple conditions, convert numbers to booleans to avoid unwanted rendering, and explicitly check for zero.
Additionally, refactor nested ternary operators into clear, separate conditions, and use early return patterns in the components to simplify complex rendering logic.
Comments ()